
Understanding Big O Notation: A Beginner's Guide
Aug 10
4 min read
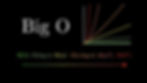
Big O Notation is a cornerstone concept in computer science, particularly in the analysis of algorithms. It provides a standardized way to describe the efficiency of algorithms by focusing on two critical aspects: time complexity and space complexity.
Understanding these concepts is crucial for anyone looking to optimize code and make informed decisions when selecting or designing algorithms.
In this blog post, we'll explore Big O Notation in detail, breaking it down into digestible parts with a simple example to illustrate its application.
What is Big O Notation?
Big O Notation is a mathematical notation used to describe the upper bound of an algorithm's time complexity or space complexity. Essentially, it helps us understand the worst-case scenario of an algorithm's performance as the input size grows.
When analyzing an algorithm, we typically ask two questions:
Time Complexity: How does the time required to execute this algorithm increase as the input size grows?
Space Complexity: How does the amount of memory the algorithm uses change as the input size grows?
Big O Notation provides a formal way to answer these questions.
Time Complexity
Time complexity refers to the amount of time an algorithm takes to run as a function of the input size. It gives us a way to estimate how an algorithm's run time will scale with increasing input.
The time complexity of an algorithm is usually expressed in Big O Notation, which represents the upper bound of the time required for an algorithm to complete. Here’s how different time complexities compare:
O(1) - Constant Time: The run time is constant, no matter how large the input is.
O(log n) - Logarithmic Time: The run time grows logarithmically as the input size increases, often seen in algorithms that divide the problem in half each step (e.g., binary search).
O(n) - Linear Time: The run time grows linearly with the input size.
O(n log n) - Log-Linear Time: The run time is a combination of linear and logarithmic growth, typical in more efficient sorting algorithms like merge sort.
O(n^2) - Quadratic Time: The run time grows quadratically with the input size, common in algorithms with nested loops.
O(2^n) - Exponential Time: The run time doubles with each additional element in the input, making the algorithm impractical for large inputs.
O(n!) - Factorial Time: The run time grows factorially, which is extremely slow and usually impractical.
Space Complexity
Space complexity refers to the amount of memory an algorithm uses in relation to the input size. Like time complexity, space complexity is also expressed using Big O Notation.
Space complexity is important because, in addition to time efficiency, an algorithm needs to be efficient in terms of memory usage, especially when dealing with large data sets or constrained environments like embedded systems.
Common space complexities include:
O(1) - Constant Space: The algorithm uses a fixed amount of memory, regardless of input size.
O(n) - Linear Space: The memory usage grows linearly with the input size.
O(n^2) - Quadratic Space: The memory usage grows quadratically with the input size.
Why is Big O Notation Important?
Big O Notation is crucial for several reasons:
Predict Performance: It helps you anticipate how your algorithm will perform as the input size increases.
Optimize Code: By understanding time and space complexities, you can choose or design algorithms that are more efficient.
Compare Algorithms: Big O Notation provides a standardized way to compare the efficiency of different algorithms, allowing you to make informed decisions.
A Simple Example: Finding the Maximum Element
Let’s take a simple example to illustrate both time and space complexity using Big O Notation. Suppose you have a list of numbers, and you want to find the largest number in the list.
Here’s a basic algorithm to accomplish this:

Start with the first number in the list as the maximum.
Compare the current maximum with each subsequent number in the list.
If a number is larger than the current maximum, update the maximum.
After going through the entire list, the maximum variable will hold the largest number.
Analyzing the Example with Big O Notation
Time Complexity:
Initialization (max_num = numbers[0]): This is an O(1) operation since it only involves assigning a value to a variable.
Looping through the list (for num in numbers): The loop runs n times if there are n numbers in the list, making this an O(n) operation.
Comparison and Update (if num > max_num): This comparison and possible update occur within the loop and thus also runs n times. Each comparison is an O(1) operation.
Overall, the time complexity of this algorithm is O(n) because the loop dominates the overall time.
Space Complexity:
Space for Variables (max_num and num): The algorithm only uses a few extra variables (e.g., max_num, num), which means it requires a constant amount of additional memory. This gives us an O(1) space complexity.
The space complexity is O(1) because the amount of memory used does not increase with the size of the input list.
Conclusion
Big O Notation is an essential tool for understanding and analyzing the efficiency of algorithms in terms of both time and space. By mastering Big O Notation, you can make better decisions when it comes to selecting or designing algorithms, ensuring that your code is both time-efficient and memory-efficient.
Whether you're dealing with simple tasks like finding the maximum element in a list or tackling more complex problems, a solid grasp of Big O Notation will help you write code that scales well and performs efficiently, even with large input sizes.